Как создать базовый чат-бот с использованием TensorFlow и JavaScript
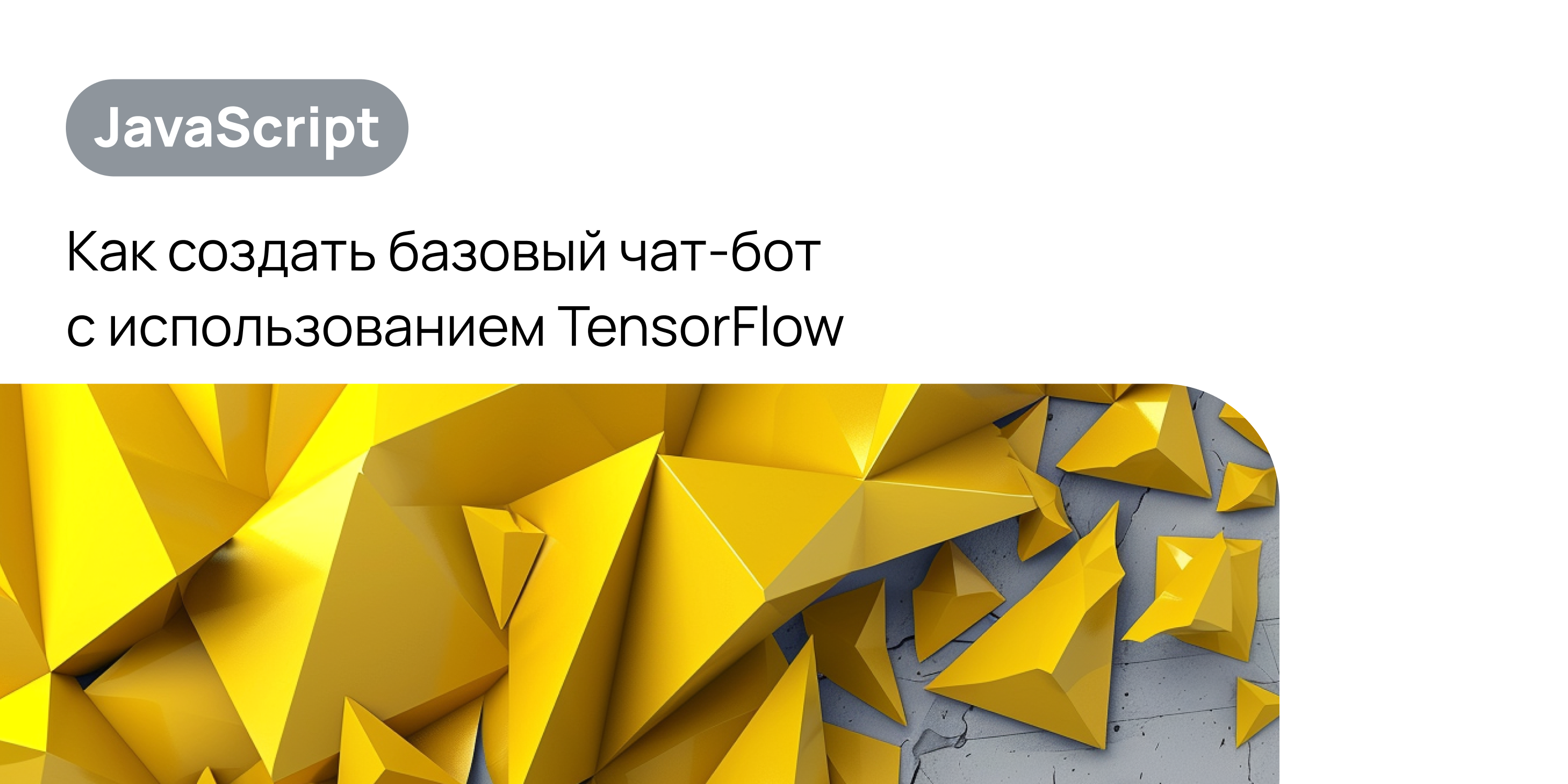
Мы все сталкиваемся с чат-ботами при посещении различных сайтов. Некоторые из них работают на основе реального человеческого взаимодействия, другие — на основе искусственного интеллекта.
В этой статье мы рассмотрим создание простого чат-бота на базе искусственного интеллекта с использованием TensorFlow и JavaScript. Чат-бот будет распознавать команды пользователя и отвечать предопределенными ответами.
Пошаговое руководство
Сначала мы создадим новый каталог для нашего проекта и инициализируем его с помощью npm
. Перед началом этого шага убедитесь, что в вашей системе установлен Node.js.
mkdir chatbot
cd chatbot
npm init -y
Установить необходимые пакеты
Для нашего простого проекта мы будем использовать следующие пакеты npm
:
tensorflow/tfjs
: библиотека TensorFlow.js для машинного обучения.tensorflow-models/universal-sentence-encoder
: предварительно обученная модель универсального кодировщика предложений для распознавания намерений.
npm install @tensorflow/tfjs @tensorflow-models/universal sentence-encoder
1. Создать intents
Создайте файл с именем intents.js
. Это категории вводимых пользователем данных, которые распознает чат-бот (например, приветствия, запросы о продуктах, статус заказа).
const intents = {
greeting: ["hello", "hi", "hey", "good morning", "good evening", "howdy"],
goodbye: ["bye", "goodbye", "see you later", "farewell", "catch you later"],
thanks: ["thank you", "thanks", "much appreciated", "thank you very much"],
product_inquiry: ["tell me about your products", "what do you sell?", "product information", "what can I buy?", "show me your products"],
order_status: ["where is my order?", "order status", "track my order", "order tracking", "order update"],
shipping_info: ["shipping information", "how do you ship?", "shipping methods", "delivery options", "how long does shipping take?"],
return_policy: ["return policy", "how to return?", "return process", "can I return?", "returns"],
payment_methods: ["payment options", "how can I pay?", "payment methods", "available payments"],
support_contact: ["contact support", "how to contact support?", "customer support contact", "support info", "customer service contact"],
business_hours: ["business hours", "working hours", "when are you open?", "opening hours", "store hours"]
};
module.exports = { intents }
2. Создать ответы
Создайте еще один файл с именем responses.js
для хранения предопределенных ответов. Это предопределенные ответы, которые чат-бот будет давать на основе распознанного намерения.
const responses = {
greeting: "Hello! How can I help you today?",
goodbye: "Goodbye! Have a great day!",
thanks: "You're welcome! If you have any other questions, feel free to ask.",
product_inquiry: "We offer a variety of products including electronics, books, clothing, and more. How can I assist you further?",
order_status: "Please provide your order ID, and I will check the status for you.",
shipping_info: "We offer various shipping methods including standard, express, and next-day delivery. Shipping times depend on the method chosen and your location.",
return_policy: "Our return policy allows you to return products within 30 days of purchase. Please visit our returns page for detailed instructions.",
payment_methods: "We accept multiple payment methods including credit/debit cards, PayPal, and bank transfers. Please choose the method that suits you best at checkout.",
support_contact: "You can contact our support team via email at support@example.com or call us at 1-800-123-4567.",
business_hours: "Our business hours are Monday to Friday, 9 AM to 5 PM. We are closed on weekends and public holidays."
};
module.exports = { responses };
3. Загрузка TensorFlow и Sentence Encoder
Создаем основной файл скрипта с именем chatbot.js
и загружаем необходимые библиотеки и модели, асинхронно загружаем модель универсального кодировщика предложений и запускаем чат-бота после загрузки модели.
const tf = require('@tensorflow/tfjs');
const use = require('@tensorflow-models/universal-sentence-encoder');
const { intents } = require('./intents');
const { responses } = require('./responses');
const readline = require('readline');
// Load the Universal Sentence Encoder model
let model;
use.load().then((loadedModel) => {
model = loadedModel;
console.log("Model loaded");
startChatbot();
});
4. Реализация распознавания намерений
Добавляем функцию распознавания намерения ввода пользователя, встраиваем ввод пользователя в многомерный вектор с помощью универсального кодировщика, а затем отслеживаем наивысшую оценку сходства на основе намерения.
async function recognizeIntent(userInput) {
const userInputEmb = await model.embed([userInput]);
let maxScore = -1;
let recognizedIntent = null;
for (const [intent, examples] of Object.entries(intents)) {
// Embedding the example phrases for each intent & Calculating similarity scores between the user input embedding and the example embeddings
const examplesEmb = await model.embed(examples);
const scores = await tf.matMul(userInputEmb, examplesEmb, false, true).data();
const maxExampleScore = Math.max(...scores);
if (maxExampleScore > maxScore) {
maxScore = maxExampleScore;
recognizedIntent = intent;
}
}
return recognizedIntent;
}
5. Генерация ответов
Добавьте функцию для генерации ответов на основе распознанного намерения:
async function generateResponse(userInput) {
const intent = await recognizeIntent(userInput);
if (intent && responses[intent]) {
return responses[intent];
} else {
return "I'm sorry, I don't understand that. Can you please rephrase?";
}
}
6. Реализация взаимодействия с чат-ботом
Наконец, реализуйте цикл взаимодействия с чат-ботом, настроив интерфейс для считывания пользовательского ввода из командной строки, запрашивая у пользователя ввод данных и генерируя соответствующие ответы:
function startChatbot() {
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
console.log("Welcome to the customer service chatbot! Type 'quit' to exit.");
rl.prompt();
rl.on('line', async (line) => {
const userInput = line.trim();
if (userInput.toLowerCase() === 'quit') {
console.log("Chatbot: Goodbye!");
rl.close();
return;
}
const response = await generateResponse(userInput);
console.log(`Chatbot: ${response}`);
rl.prompt();
});
}
Вот готовый код для chatbot.js
:
const tf = require('@tensorflow/tfjs');
const use = require('@tensorflow-models/universal-sentence-encoder');
const { intents } = require('./intents');
const { responses } = require('./responses');
const readline = require('readline');
// Load the Universal Sentence Encoder model
let model;
use.load().then((loadedModel) => {
model = loadedModel;
console.log("Model loaded");
startChatbot();
});
async function recognizeIntent(userInput) {
const userInputEmb = await model.embed([userInput]);
let maxScore = -1;
let recognizedIntent = null;
for (const [intent, examples] of Object.entries(intents)) {
const examplesEmb = await model.embed(examples);
const scores = await tf.matMul(userInputEmb, examplesEmb, false, true).data();
const maxExampleScore = Math.max(...scores);
if (maxExampleScore > maxScore) {
maxScore = maxExampleScore;
recognizedIntent = intent;
}
}
return recognizedIntent;
}
async function generateResponse(userInput) {
const intent = await recognizeIntent(userInput);
if (intent && responses[intent]) {
return responses[intent];
} else {
return "I'm sorry, I don't understand that. Can you please rephrase?";
}
}
function startChatbot() {
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
console.log("Welcome to the customer service chatbot! Type 'quit' to exit.");
rl.prompt();
rl.on('line', async (line) => {
const userInput = line.trim();
if (userInput.toLowerCase() === 'quit') {
console.log("Chatbot: Goodbye!");
rl.close();
return;
}
const response = await generateResponse(userInput);
console.log(`Chatbot: ${response}`);
rl.prompt();
});
}
7. Для запуска чат-бота выполните chatbot.js файл:
node chatbot.js
Вуаля! В результате выполнения нашей команды чат-бот должен запуститься:
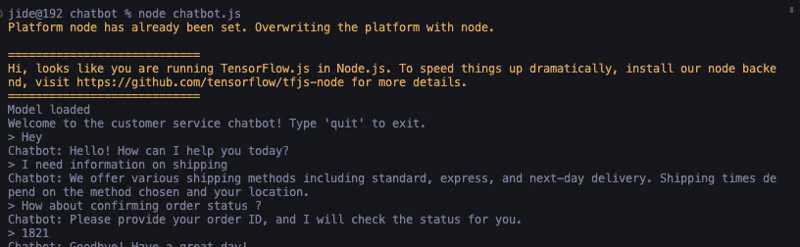
Заключение
В этой статье мы создали простой чат-бот для обслуживания клиентов с использованием TensorFlow и JavaScript. Хотя эта реализация является базовой, она обеспечивает прочную основу для создания более сложных чат-ботов. Вы можете расширить этот проект, интегрировав API с помощью AXIOS, добавив больше намерений и ответов или развернув его на веб-платформе.