07.03.2024 в 16:33
Полина Родионова
Стилизация псевдоэлемента CSS с помощью JavaScript
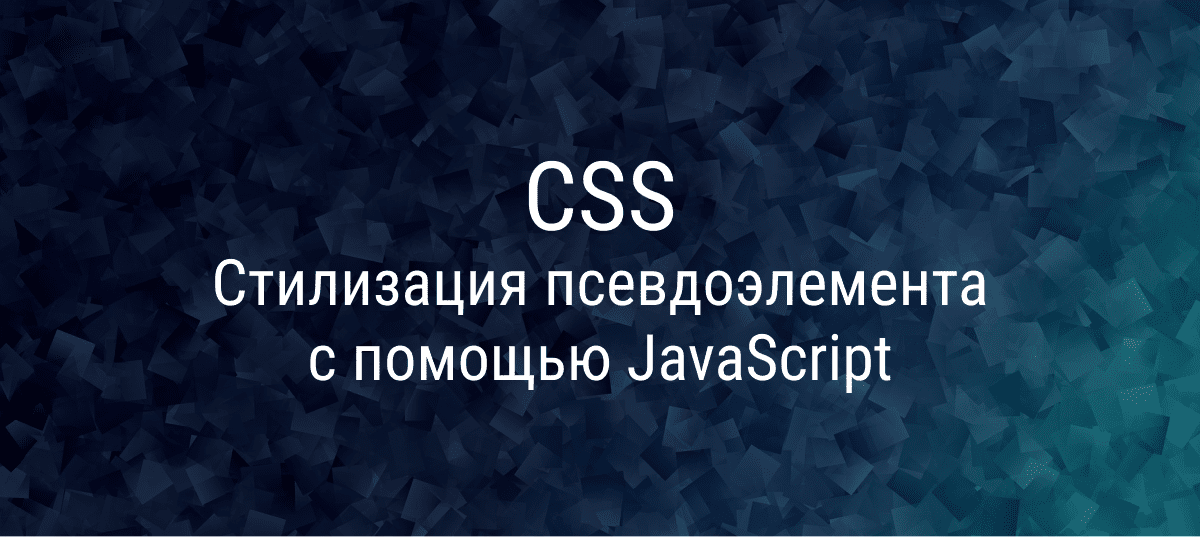
В JavaScript вы не сможете сделать какой-нибудь селектор запросов, например:
document.querySelector("div::after");
Но с помощью переменных CSS вы можете изменять стили этих селекторов с помощью JavaScript!
В CSS выберите имя переменной и присвойте его чему-нибудь:
div::after {
/* 50px is the default value if --somewidth doesn't exist */
width: var(--somewidth, 50px);
}
А в JavaScript используйте функцию setProperty
, чтобы присвоить значение этой переменной!
// this is just grabbing a div, you can change it to select any element
const element = document.getElementsByTagName("div")[0];
element.style.setProperty("--somewidth", "50%");
Ну вот и всё! При необходимости вы можете усложнить этот процесс. Обратите внимание на строку 38 в CSS и строку 25 в JavaScript!
<h1>My Goal: $5,000</h1> <!-- Update this -->
<p>Currently at <span id="status">0%</span> of our goal!</p>
<div id="amount"></div>
<p>Latest activity: <span id="activity">wee</span></p>
html {
font-family: 'Helvetica Neue', 'Helvetica', 'Arial', sans-serif;
font-size: 1.3em;
max-width: 40rem;
padding: 2rem;
margin: auto;
line-height: 1.5rem;
}
div {
position: relative;
margin: 10px auto;
width: clamp(320px, 100%, 500px);
height: 100px;
border: 5px solid black;
border-radius: 100px;
overflow: hidden;
}
div::before {
display: block;
content: '';
width: 100%;
height: 50%;
background-color: #ffffff;
opacity: 1;
background-size: 50px 50px;
background-image: repeating-linear-gradient(to right, #000000, #000000 2px, rgba(255,255,255,0) 2px, rgba(255,255,255,0));
z-index: -1;
}
div::after {
display: block;
content: '';
position: absolute;
bottom: 0;
background: rgba(228, 0, 43, .9);
width: var(--perc, auto);
height: 100px;
}
span {
font-weight: bold;
}
// Add activities here (to the top) that have an amount
let activity = [
{ name: 'Conference talk', amount: '1500' },
{ name: 'Etsy sale', amount: '20' },
{ name: 'This is an example', amount: '250' },
];
// Update your goal amount here
const goalAmount = 5000;
// You can ignore the below
function updateAmount() {
const maxAmount = goalAmount;
let totalAmount = 0;
for (let i = 0; i < activity.length; i++) {
totalAmount += parseInt(activity[i].amount, 10);
}
const percentage = (totalAmount / maxAmount) * 100;
const amountDiv = document.getElementById('amount');
const statusDiv = document.getElementById('status');
const activityDiv = document.getElementById('activity');
amountDiv.style.setProperty('--perc', percentage + '%');
statusDiv.textContent = `$${totalAmount}, or ${Math.ceil(percentage)}%,`;
activityDiv.textContent = activity[0].name;
}
updateAmount();